How to process JSON/XML string in SAP Cloud Applications Studio? – Part 2
In the last part, we presented how to implement a simple SOAP service in Java. This part of the series will aim to extend our simple SOAP service by calling the REST service and thus obtaining data from the REST service and their subsequent processing into the SOAP service. We will also complete the implementation that needs to be done in Java.
In this part, we will use the code created in the previous part (you can download it there). We will extend it with classes and methods that implement calls to the REST service and the processing of acquired data. For example, we decided to use the freely available REST service, which obtains data on the development of Covid-19 in the world. Documentation for this service is available at:
To download the soap guide
Implementation
In package types, we create two new classes, one to describe the structure of the data we want to send to the output and the other to store this data in the letter. We will call our classes CountryData and CountryDataList. The implementation of these classes is shown in the examples below:
package types; import java.util.Calendar; public class CountryData { private String Country; private int Confirmed, Dead, Recovered; private Calendar Updated; public String getCountry() { return Country; } public void setCountry(String country) { Country = country; } public int getConfirmed() { return Confirmed; } public void setConfirmed(int confirmed) { Confirmed = confirmed; } public int getDead() { return Dead; } public void setDead(int dead) { Dead = dead; } public int getRecovered() { return Recovered; } public void setRecovered(int recovered) { Recovered = recovered; } public Calendar getUpdated() { return Updated; } public void setUpdated(Calendar updated) { Updated = updated; } }
package types; import java.util.List; public class CountryDataList { private List CountryDataList; public List getCountryDataList() { return CountryDataList; } public void setCountryDataList(List countryDataList) { CountryDataList = countryDataList; } }
We will create a new package in the Java folder and name it utils. This package will create two new Java classes called ApiCalls and DataParser. The API calls class will include implementing a method for obtaining responses from the services used. The content of the API calls method is shown in the code sample below:
package utils; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class ApiCalls { public static String getResponse(URL url, String restMethod) throws IOException { String response = ""; HttpURLConnection con = null; con = (HttpURLConnection) url.openConnection(); con.setRequestMethod(restMethod); int status = con.getResponseCode(); if(status == 200) { BufferedReader in = new BufferedReader( new InputStreamReader(con.getInputStream())); String inputLine; while ((inputLine = in.readLine()) != null) { response += inputLine; } in.close(); } con.disconnect(); return response; } }
Before the next step, you need to edit the pom.xml file in the root directory and add the following dependency to the dependencies:
<dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>2.4</version> </dependency>
In the DataParser method, we implement the data processing logic we received when calling the REST service. The contents of the DataParser method are illustrated by the following code sample:
package utils; import com.google.gson.JsonArray; import com.google.gson.JsonElement; import com.google.gson.JsonObject; import com.google.gson.JsonParser; import types.CountryData; import java.io.IOException; import java.net.MalformedURLException; import java.net.URL; import java.time.ZonedDateTime; import java.util.ArrayList; import java.util.GregorianCalendar; import java.util.List; public class DataParser { public static List getCountryDataList() { List CountryDataList = new ArrayList<>(); String serviceResponse = callService("https://www.trackcorona.live/api/countries", "GET"); if (serviceResponse != null) { JsonArray JsonRespArr; JsonObject JsonObjectResp; JsonElement JsonElementResp = new JsonParser().parse(serviceResponse); if (JsonElementResp.getAsJsonObject() != null) { JsonObjectResp = JsonElementResp.getAsJsonObject(); if (JsonObjectResp.has("data")) { JsonRespArr = JsonObjectResp.getAsJsonArray("data"); for (JsonElement Item : JsonRespArr ) { CountryDataList.add(getDataForCountry(Item)); } } } } return CountryDataList; } public static CountryData getDataForCountry(JsonElement Item) { CountryData data = new CountryData(); JsonObject DataObj = Item.getAsJsonObject(); data.setCountry(DataObj.get("location").getAsString()); data.setUpdated(GregorianCalendar.from(ZonedDateTime.parse(DataObj.get("updated").getAsString().replace(" ", "T")))); data.setConfirmed(DataObj.get("confirmed").getAsInt()); data.setDead(DataObj.get("dead").getAsInt()); data.setRecovered(DataObj.get("recovered").getAsInt()); return data; } public static String callService(String Url, String restMethod) { URL url = null; try { url = new URL(Url); } catch (MalformedURLException e) { e.printStackTrace(); } String response = null; try { response = ApiCalls.getResponse(url, restMethod); } catch (IOException e) { e.printStackTrace(); } return response; } }
We modify the GuideService class, to which we add the following method:
@WebMethod(operationName = "getCountryDataList") public CountryDataList getCountryDataList() { CountryDataList countryDataList = new CountryDataList(); countryDataList.setCountryDataList(DataParser.getCountryDataList()); return countryDataList; }
Finally, it is necessary to regenerate the war file using the command “mvn package” – the detailed procedure was described in the previous section. The project must be re-uploaded to the SAP Cloud Platform, and we recommend uploading the application with a new name.
The result of this part of the blog is a SOAP service that reads and processes data from the REST service and provides output in SOAP format.
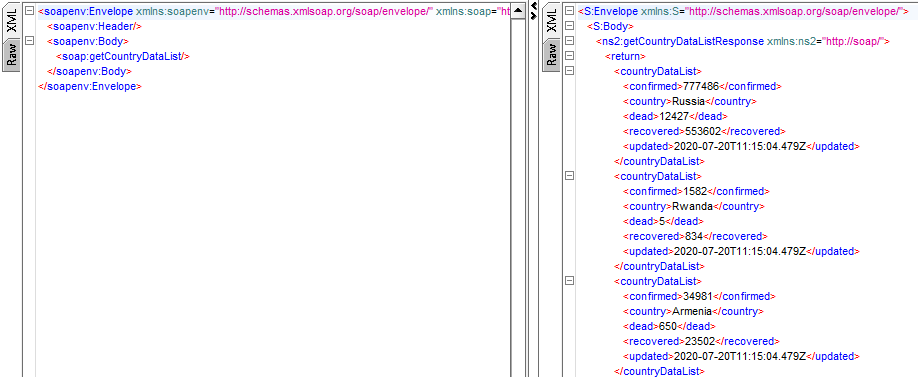
In the following part of the series, we will show you how to use our SOAP service when creating objects in SAP Cloud for Customers with the help of Application Studio.